HELP
B. The names of the picked assets will be listed in the code comments.
C. Use the assets with new Pic("name") or new Aud("name"). SEE VIDEO
D. Scroll down and press the wands below to see helpful examples!
To change the background color you can use the color property of the frame (F)
// use the color property of the frame for the stage color
F.color = yellow; // F is the frame!
// use the outerColor property for the color outside the stage
F.outerColor = blue;
// use a Page to make a gradient
new Page(W, H, green, pink).addTo();
We will add a picture of a BEACH for a background picture.
steps to add picture
- Press the ASSETS button at the top of the page
- Then press the BACKINGS button at the top
- Select and save the "beach01" picture
- You should see "Backings: beach01" in the comments
- Use the code below to put the asset on the stage
// ** DO THE STEPS ABOVE FIRST ** // A background picture can be scaled to the stage // then center() and mov(x, y) or loc(x, y) can be used new Pic("beach01") .scaleTo() .center();
Let's add a picture of a BUTTERFLY and make it draggable!
steps to add picture
- Press the ASSETS button at the top of the page
- Then press the NATURE button at the top
- Select and save the "bug01" picture
- You should see "Nature: bug01" in the comments
- Use the code below to put the asset on the stage
// pictures can be scaled with sca() if you want // sca(.5) is half as big, sca(2) is twice as big // You can use center() and mov(x, y) or // loc(x, y) or pos(x, y, RIGHT, BOTTOM), etc. // Store the bug in a variable to use later with sounds const bug = new Pic("bug01") .sca(.5) .center() .drag();
Emojis are fun! We can easily add Emojis using the ZIM Emoji() class:
// add an Emoji // inside "", use WINDOW KEY . to pick an emoji // or use Control + Command + Space on a Mac // choose a number for the size like 100 new Emoji("🐞", 100).center().drag();
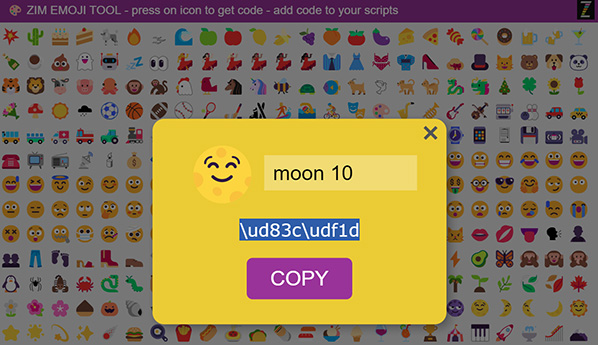
You can also copy the code from the ZIM Emoji Picker
// add an Emoji with an Emoji Code // go to the Emoji Picker and press an Emoji // then press COPY and paste the code in the "" new Emoji("\ud83c\udf1d", 200).loc(100,100).drag();
Sometimes we want to group our objects so they move together.
Let's put "gem01" and "cartoon02" in a Container and drag them.
steps to add pictures
- Press the ASSETS button at the top of the page
- Select and save the "gem01" picture from NATURE assets
- Select and save the "cartoon02" picture from PEOPLE assets
- You should see the pictures listed in the comments
- Use the code below to put the pictures in a Container
// make a Container (an invisible holder) const holder = new Container(); // add the gem new Pic("gem01").addTo(holder); // scale the cartoon face to FIT in 60% the holder width // and center it in the container new Pic("cartoon02") .scaleTo(holder, 60) // 60% the size .center(holder); // position the container and drag all of it together holder .pos(100,50,RIGHT,BOTTOM) .drag({all:true});
Here we play a POWERUP sound when we press the butterfly!
steps to add sound
- Press the ASSETS button at the top of the page
- Then press the SOUNDS button at the top
- Select and save the "powerup" sound
- You should see "Sounds: powerup" in the comments
- Use the code below play the sound when pressing the butterfly
// To play a sound when pressing the bug (from above) // use an event and play the sound in the event function // Aud is short form for Audio - another way to say sound! const sound = new Aud("powerup"); // make the sound once bug.on("mousedown", ()=>{ sound.play(); // play the sound every time we press the bug });
Let's play some BACKING music. We show four ways to play a background sound because sounds can only be played after the first mousedown.
steps to add sound
- Press the ASSETS button at the top of the page
- Then press the SOUNDS button at the top
- Select and save the "backing" sound
- From wand 5 you may already have a powerup sound
- So you should see "Sounds: backing, powerup" in the comments
- Use ONE of the examples below to play the background sound
// ** OPTION ONE - BASIC ** // This code works when we TEST to play a backing sound // but will not work when we use FULL and SAVE - boo! // Here we lower the volume and loop the backing sound. new Aud("backing").play(.1, true);
// ** OPTION TWO - PANE ** // This code works in TEST, FULL and SAVE. Yay! // Here we play the backing sound once a Pane() is closed. new Pane("START", yellow).show(()=>{ new Aud("backing").play(.1, true); });![]()
// ** OPTION THREE - TOGGLE ** // This code lets people turn on and off the sound. // Do not worry if this is complicated looking // it will just help you pause or play the sound. let backingSound; const toggle = new Toggle({ label:"music", startToggled:false }) .sca(.7) .pos(40,40,LEFT,BOTTOM) .change(()=>{ if (!backingSound) { backingSound = new Aud("backing").play(.1, true); } backingSound.paused = !toggle.toggled; });![]()
// ** OPTION FOUR - BUTTON ** // This code uses a Button to toggle sound. // We use pizzazz to make sound icons // or you could use label:"play" and toggle:"mute" // We use the tap() method which is like on("click", function). let backingSound; const button = new Button({ width:60, height:60, corner:0, backgroundColor:purple, rollBackgroundColor:pink, icon:makeIcon("sound", white), toggleIcon:makeIcon("mute", white) }).pos(50,50,LEFT,BOTTOM).tap(()=>{ if (!backingSound) { backingSound = new Aud("backing").play(.1, true); } backingSound.paused = !button.toggled; });![]()
A Sprite() is made from a SpriteSheet which is a grid of pictures. There are TWO types:
1. DIFFERENT OBJECTS
Found in the AI images. You can run() a single frame to show the image you want.
steps to add a sprite
- Press the ASSETS button at the top of the page
- Then press the AI button at the top
- Select and save the "ai_backpacks" picture
- You should see "AI: ai_backpacks" in the comments
- Use the code below to show a backpack
// A Sprite() to show different objects. // On the menu page, they have a dashed border // with COLS and ROWS in black // press the purple arrow to see a preview new Sprite("ai_backpacks", 5, 2) // 5 columns and 2 rows .center() .run({frame:1}); // show the second backpack (0 is the first) // or Emit a bunch of backpacks! new Emitter(new Sprite("ai_backpacks", 5, 2)).center();
2. ANIMATIONS
An animation Sprite() uses pictures of an object in motion. There are a few animation sprites (not in AI). Here is a Video about Sprites
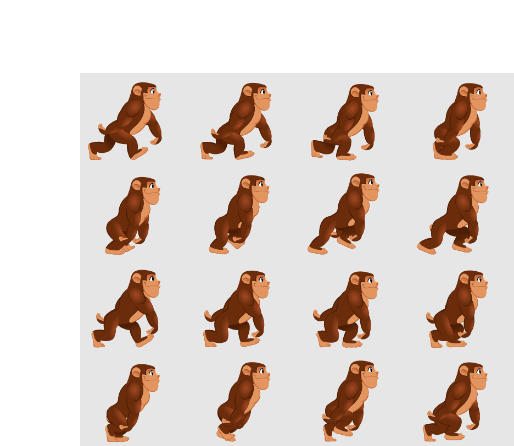
steps to add a sprite
- Press the ASSETS button at the top of the page
- Then press the NATURE button at the top
- Select and save the "monkey" picture
- You should see "Nature: monkey" in the comments
- Use the code below to animate the sprite
// The Sprite is made from a SpriteSheet. // On the menu page, they have a dashed border // with COLS and ROWS in black // press the purple arrow to see a preview // We must run() the sprite as well // which is like animate() but for Sprites. // The longer the time the slower the animation. new Sprite("monkey", 4, 4) .center() .run({time:1, loop:true});
Pictures, Sounds and Sprites can be loaded from the Internet. There may be permission issues so talk to your leader.
// Here we load from the ZIM assets folder. // See assets: https://zimjs.org/assets/ PATH = "https://zimjs.org/assets/"; // tell ZIM where the assets are new Pic("nature03.jpg") .scaleTo() .center(); const start = new Aud("start.mp3"); const bike = new Pic("transport03.png") .reg(CENTER,BOTTOM) .pos(0,100,CENTER,BOTTOM) .tap(()=>{ start.play(); bike.animate({x:-200}, 3, "backIn"); });
// For a Sprite, we have to preload the asset // We normally load assets in the ZIM Frame() // but the Frame is already made in Slate // so instead, we can use loadAssets() // this sprite is packed so needs json data F.loadAssets(["spaceguy.png", "spaceguy.json"], "https://zimjs.org/assets/"); F.on("complete", ()=>{ new Sprite({json:"spaceguy.json"}) .sca(2) .center() .run({loop:true}); }); // See sidescroller examples with mouse: // Sidescroller with Mouse // with keyboard and shooting: // Sidescroller with Keys